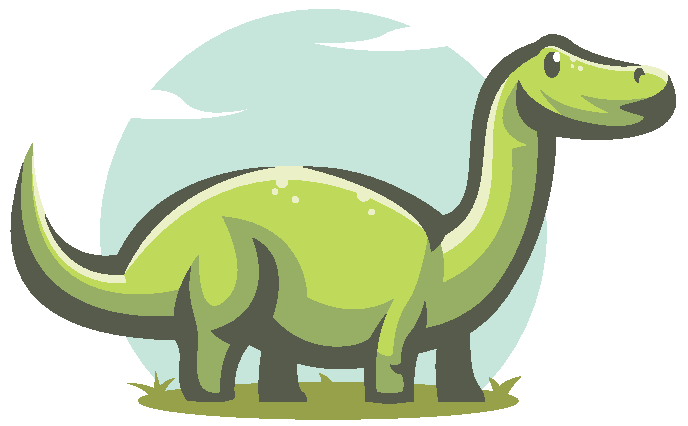
Components to Markdown
Highly customizable open source tool for generating component documentation.
Custom Templates
Create your own template and customize all the content. Or choose from our templates, designed to be intuitive, responsive and with dark mode support.
Multi-Framework Support
Markdown is the language used by most frameworks, like Docusaurus and Storybook for example. Or use it directly in your git repository.
Use as CLI or library
Generate all documentation with just one command, or extend its functionality by importing it into your JavaScript/TypeScript code.
How it works
1. Write Your Component
You will develop your code in the conventional way using types:
import React, { ReactNode } from 'react';
type Variant = 'default' | 'primary' | 'success' | 'danger';
export interface ButtonProps {
/**
* The text to display in the button.
*/
children: ReactNode;
/**
* The button type.
*
* @defaultValue `'button'`
*/
type?: 'button' | 'submit' | 'reset';
/**
* The button variant.
*
* @since 1.1.0
* @defaultValue `'default'`
*/
variant?: Variant;
/**
* Button click event handler.
*
* @example Logging the click event
* ```tsx
* onClick={() => {
* console.log('Button clicked');
* }}
* ```
*
* @param event The click event.
* @returns void
*/
onClick?: (event: React.MouseEvent<HTMLButtonElement>) => void;
}
/**
* Buttons allow users to take actions, and make choices, with a single tap.
*
* @remarks
* Buttons communicate actions that users can take.
* They are typically placed throughout your UI, in places like:
* - Dialogs
* - Modal windows
* - Forms
* - Cards
* -Toolbars
*
* @beta
* @public
*/
export function Button({ children, onClick, type = 'button' }: ButtonProps) {
return (
<button type={type} onClick={onClick}>
{children}
</button>
);
}
2. Choose a template
Then you can create your own template or select one of the available templates:
3. Generate the Documentation
Now just generate your awesome documentation.
Below is a demo according to the template chosen above:
Button
Beta Public
Buttons allow users to take actions, and make choices, with a single tap.
Remarks
Buttons communicate actions that users can take.
They are typically placed throughout your UI, in places like:
- Dialogs
- Modal windows
- Forms
- Cards -Toolbars
Properties
children
✳️
The text to display in the button.
ReactNode;
type
The button type.
Default Value: 'button'
'button' | 'submit' | 'reset';
variant
The button variant.
Since: 1.1.0
Default Value: 'default'
'default' | 'primary' | 'success' | 'danger';
onClick
Button click event handler.
(event: React.MouseEvent<HTMLButtonElement>) => void
Parameters
Name | Description |
---|---|
event | The click event. |
Returns
void
Examples
Logging the click event
onClick={() => {
console.log('Button clicked');
}}